#Python
Different ways to reverse String in Python.
String is a widely used datatype in Python just like a list. In most of the interviews, a question was asked How to reverse a string in Python?
In this article, we will see a different way to reverse a String in Python.
1. Using Extended Slice Syntax:
Extended slice method takes three parameters [ start index: end index: increment ] just like the for a loop.
Example:
testString = 'Neptuneworld' testString=testString[::-1] print(testString) |
Output:
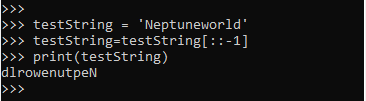
2.Using BuildIn method Reversed():
Reversed method returns a string reversed object which we can see that’s why we use to join and convert that object to a string using join.
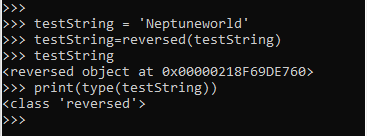
Example :
testString = 'Neptuneworld' testString=””.join(reversed(testString)) print(testString) |
Output :
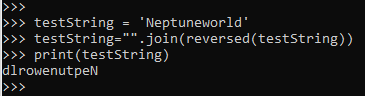
3. Using for loop:
In this method, we iterate the string and add the element to the start of initialized empty string.
Example:
testString = 'Neptuneworld' revString = str() for ch in testString: revString = ch + revString print(revString) |
Output:

4.Using Recursive Function:
def reverse(testString): if len(testString) == 0: return testString else: return reverse(testString[1:]) + testString[0] print(reverse("NeptuneWorld")) |
5.Using List :
Using list also we can reverse the string as shown below.
testString = 'Neptuneworld' revString = [] for i in range(len(testString)-1,-1,-1): revString.append(testString[i])
print(''.join(revString)) |
Thanks for Reading !!!